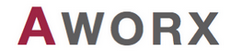 |
ALib C++ Library
|
Library Version: 2312 R0
Documentation generated by
|
10 # if !defined (HPP_ALIB_FS_MODULES_MODULE)
13 # if !defined(HPP_ALIB_FS_MODULES_DISTRIBUTION)
17 # if ALIB_BOXING && !defined (HPP_ALIB_BOXING_BOXING)
21 # if ALIB_CONFIGURATION
22 # if !defined (HPP_ALIB_CONFIG_CONFI)
25 # if !defined (HPP_ALIB_CONFIG_CONFIGURATION)
30 # if !defined (HPP_ALIB_ENUMS_ITERABLE)
34 # if !defined (HPP_ALIB_RESOURCES_LOCALRESOURCEPOOL)
39 # if !defined (HPP_ALIB_TIME_TIME)
44 # if ALIB_THREADS && !defined (HPP_ALIB_THREADS_THREAD)
49 # if !defined (_GLIBCXX_IOSTREAM) && !defined (_IOSTREAM_ )
52 # if !defined (_GLIBCXX_IOMANIP) && !defined (_IOMANIP_ )
55 #endif // !defined(ALIB_DOX)
59 namespace aworx {
namespace lib {
81 "Command line args ignored. "
82 "Accepted only with initialization level 'PrepareResources'." )
86 ALIB_WARNING(
"MODULE",
"Given initialization level already performed on module. "
94 *thisModuleIt != this )
98 "Trying to bootstrap a module that is not included in list ALibDistribution::Modules.\n"
99 "Resource category of the module: ", (*thisModuleIt)->ResourceCategory )
130 hashMap.MaxLoadFactor ( 5.0 );
137 for(
auto moduleIt= thisModuleIt ; moduleIt !=
ALIB.
Modules.rend() ; ++moduleIt )
140 if( (*moduleIt)->resourcePool !=
nullptr && (*moduleIt)->resourcePool != actPool)
142 actPool= (*moduleIt)->resourcePool;
146 (*moduleIt)->resourcePool= actPool;
152 #if !ALIB_CONFIGURATION
153 (void) argc; (void) argvN; (void) argvW;
183 if ( argvN !=
nullptr )
192 for(
auto module= thisModuleIt ; module !=
ALIB.
Modules.rend() ; ++module )
195 if( (*module)->config !=
nullptr && (*module)->config != actConfig)
197 actConfig= (*module)->config;
201 (*module)->config= actConfig;
208 ALIB_DBG(
bool foundThisModuleInList =
false; )
217 module->resourcePool ==
nullptr )
221 #if ALIB_CONFIGURATION
223 module->config ==
nullptr )
230 module->bootstrap( actualPhase, argc, argvN, argvW );
235 if ( module ==
this )
237 ALIB_DBG( foundThisModuleInList =
true );
242 "The module that method Bootstrap was invoked on is not included in "
243 "list Module::BootstrapModules." )
255 ALIB_WARNING(
"MODULE",
"Termination level already performed." )
272 bool foundThisModule=
false;
278 if ( !foundThisModule )
280 if ( *module !=
this )
282 foundThisModule =
true;
287 || ( *module )->bootstrapState < 0,
"MODULES",
288 "Trying to terminate a not (fully) initialized module. "
294 ( *module )->shutdown( actualPhase );
304 #if ALIB_CONFIGURATION
361 std::cout << std::left << std::setw(30) <<
"Symbol" <<
'|' << std::setw(5) <<
" Lib" <<
'|' <<
" Comp. Unit" << std::endl;
365 std::cout << std::setw(30) << p.first <<
'|' << std::setw(5) << (
CompilationFlags & p.second ?
" On" :
" Off")
366 <<
"|" << std::setw(5) << (flags & p.second ?
" On" :
" Off")
constexpr std::underlying_type< TEnum >::type UnderlyingIntegral(TEnum element) noexcept(true)
ALIB_FORCE_INLINE T * Emplace(TArgs &&... args)
#define ALIB_IF_THREADS(...)
The main phase of termination that destructs everything.
resources::ResourcePool * resourcePool
platform_specific integer
#define ALIB_WARNING(...)
ALIB_API void BootstrapFillDefaultModuleList()
Keeps responsibility, e.g. when passing an object.
MonoAllocator GlobalAllocator(8 *1024)
#define ALIB_IF_ALOX(...)
void ReleaseGlobalAllocator()
NCString ResourceCategory
config::Configuration * config
ALIB_API bool Bootstrap(BootstrapPhases targetPhase=BootstrapPhases::Final, int argc=0, const char **argvN=nullptr, const wchar_t **argvW=nullptr)
#define ALIB_IF_EXPRESSIONS(...)
ALIB_API void Shutdown(ShutdownPhases targetPhase=ShutdownPhases::Destruct)
bool RemovePlugin(TPlugin *plugIn)
#define ALIB_IF_SINGLETONS(...)
MonoAllocator & AcquireGlobalAllocator(const NCString &dbgFile, int dbgLine, const NCString &dbgFunc)
TPluginType * GetPluginTypeSafe(TPriorities priority)
#define ALIB_IF_TIME(...)
#define ALIB_IF_TEXT(...)
lib::ALibDistribution ALIB
The final initialization phase.
detail::StaticResourceMap & BootstrapGetInternalHashMap()
#define ALIB_CALLER_PRUNED
#define ALIB_IF_RESULTS(...)
void InsertPlugin(TPlugin *plugin, TPriorities priority, Responsibility responsibility=Responsibility::KeepWithSender)
#define ALIB_ASSERT_ERROR(cond,...)
ALIB_API bool VerifyCompilationFlags(uint64_t compilationFlags)
#define ALIB_IF_CONFIGURATION(...)
#define ALIB_ASSERT_WARNING(cond,...)
std::vector< std::pair< const nchar *, uint64_t > > CompilationFlagMeanings
Referring to an absolute value.
static ALIB_FORCE_INLINE void Destruct(T *object)
static bool microModulesInitialized
void BaseLoadFactor(float newBaseLoadFactor)
#define ALIB_IF_BOXING(...)
void SetCommandLineArgs(int argc=0, const nchar **argv=nullptr)
const uint64_t CompilationFlags
#define ALIB_IF_SYSTEM(...)