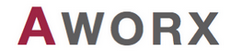 |
ALib C++ Library
|
Library Version: 2312 R0
Documentation generated by
|
Go to the documentation of this file.
8 #ifndef HPP_ALIB_FS_MODULES_MODULE
9 #define HPP_ALIB_FS_MODULES_MODULE 1
11 #if !defined(HPP_ALIB_STRINGS_CSTRING)
15 #if !defined (HPP_ALIB_RESOURCES_RESOURCES)
19 #if !defined (HPP_ALIB_ENUMS_UNDERLYING_INTEGRAL)
26 #if !defined (_GLIBCXX_ALGORITHM) && !defined(_ALGORITHM_)
29 #if !defined (_GLIBCXX_VECTOR) && !defined(_VECTOR_)
32 #if !defined (_GLIBCXX_LIST) && !defined(_LIST_)
124 #if ALIB_CONFIGURATION
146 #endif // ALIB_CONFIGURATION
207 uint64_t compilationFlags = 0 )
234 "Destructing a non-terminated module. "
296 const char** argvN =
nullptr,
297 const wchar_t** argvW =
nullptr );
299 #if defined(ALIB_DOX)
313 template<
typename TChar>
316 template<
typename TChar>
321 if( std::is_same<
typename std::remove_const<TChar>::type,
char>::value )
323 reinterpret_cast<const char **>(const_cast<const TChar**>(argv)),
326 return Bootstrap( targetPhase, argc,
nullptr,
327 reinterpret_cast<const wchar_t**>(const_cast<const TChar**>(argv)));
371 #if ALIB_CONFIGURATION
393 "This module already has a configuration object set." )
427 "A custom resource pool must be set prior to module initialization.")
531 int argc,
const char** argv,
const wchar_t** wargv ) = 0;
553 #endif // HPP_ALIB_FS_MODULES_MODULE
constexpr std::underlying_type< TEnum >::type UnderlyingIntegral(TEnum element) noexcept(true)
The main phase of termination that destructs everything.
resources::ResourcePool * resourcePool
#define ATMP_T_IF(T, Cond)
#define ALIB_ASSERT_FILESET(filesetname)
virtual void shutdown(ShutdownPhases phase)=0
const String & TryResource(const NString &name)
void operator=(const Module &)=delete
Module(int version, int revision, const NCString &resourceCategory, uint64_t compilationFlags=0)
NCString ResourceCategory
virtual void bootstrap(BootstrapPhases phase, int argc, const char **argv, const wchar_t **wargv)=0
virtual const String & Get(const NString &category, const NString &name, bool dbgAssert)=0
config::Configuration * config
ALIB_API bool Bootstrap(BootstrapPhases targetPhase=BootstrapPhases::Final, int argc=0, const char **argvN=nullptr, const wchar_t **argvW=nullptr)
#define ATMP_EQ( T, TEqual)
ALIB_API void Shutdown(ShutdownPhases targetPhase=ShutdownPhases::Destruct)
void BootstrapResource(const NString &name, const String &data)
The final initialization phase.
config::Configuration & GetConfig()
#define ALIB_ASSERT_ERROR(cond,...)
lib::config::Configuration Configuration
Type alias in namespace aworx.
ALIB_API bool VerifyCompilationFlags(uint64_t compilationFlags)
#define ALIB_ASSERT_WARNING(cond,...)
std::vector< std::pair< const nchar *, uint64_t > > CompilationFlagMeanings
void BootstrapSetResourcePool(resources::ResourcePool *pool)
static bool microModulesInitialized
void BootstrapSetConfig(config::Configuration *pConfig)
lib::resources::ResourcePool ResourcePool
Type alias in namespace aworx.
const uint64_t CompilationFlags
void Bootstrap(const NString &category, const NString &name, const String &data)
const String & GetResource(const NString &name)
ResourcePool & GetResourcePool()